CPSC 111 2005/6 Winter Term 2, Assignment 1: Lotteries and Magicians
Contents: Details | Part 1 |
Part 2 | Deliverables
Out: Tue 17 Jan 2006
Due: Tue Jan 31 2006, 5:00pm, both hard copy and electronic
handin
Value: 4% of final grade (subject to instructor discretion)
Objectives:
Note: Each assignment (and usually
each part of each assignment) will include reflection questions. You
should include your answers to the reflection questions in a separate text file from your code named README.txt.
Please do not submit your questions in any format other than plain
text. (Note that neither Microsoft Word nor PDF is plain text! Whatever
editor you use to write your source code should be usable to write a
plain text README.txt file.) You should answer the reflection questions for all parts of an assignment in the same README.txt file.
In this part of the assignment you are given a Java program that
contains both syntax and logic errors. Your task is to identify and
correct each of these errors. You will also learn a useful trick for
helping to debug your programs.
Start by downloading the source file LotteryWinnings.java.
This source file contains a Java program that helps the fictitious lottery CPSC49 Prize organizers determine ticket cost.
Specification:
- The pricing works as follows:
- every 10th ticket will be free. That is, you can buy 10
tickets for the price of 9, 20 tickets for the price of 18,
and so forth.
- in addition to the base cost of a ticket, PST (at 7.5%) and
GST (at 7%) are payable on the full amount
- The program must prompt the user for the following data:
- the starting money a player has
- the base cost of a ticket (before tax)
- the number of tickets to purchase
- The program must then print out:
- the worst case outcome
- the expected outcome: the average amount the user wins if
you imagine the user playing the same "game" (starting money,
number of tickets, price of tickets, odds of winning, size of
prize) MANY MANY times. The expected winnings are the odds of winning
times the prize money times the number of tickets played.
- the best case outcome
- The CPSC49 Prize is worth $20 million. To win it, you must
select six numbers each from 1-49 and match all six drawn in the
lottery draw.
Your task in this part of the assignment is to hand in a corrected version of the source code.
Reflection Questions:
Be sure to answer these reflection questions about Part 1 after you
finish the part. There are no right answers to these questions, and we
don't expect more than a couple of sentences for each question.
However, we will grade on your effort to answer thoughtfully.
- Which kind of error was harder to find and fix: syntax or logical errors? Why?
- Pick one logical error you found and propose a way that you
could write code to help you avoid ever making that error in the first
place. Would you rather use your technique or debug errors after
they're made?
- OPTIONAL: What was especially interesting or
frustrating about this part of the assignment? What could we have done
to make it better for you?
Hints:
- How do I detect syntax errors?
The Java compiler does a great job of detecting syntax errors! As it
tries to translate the code, it will print an error message when it
finds a statement that it cannot translate. The Java compiler will
often suggest what might be wrong (e.g. ";" missing, or
")" missing). So, to detect syntax errors, simply try to
compile your code. Take note of any errors and correct them. Each time you fix a syntax error, recompile your
code. Keep doing this until there are no syntax errors.
Usually, it's best to start with the first error Java
reports after each compilation! Often the later errors are reported
just because Java became confused by the first error.
Also note that Java's error messages are not always entirely
correct or helpful!
- How do I detect logic errors?
Logic errors are much more difficult to find than syntax errors. The compiler
is no use to you here. When testing a program, your goal is to detect logic
errors. It's like reading a good murder mystery. Put your detective hat on,
think critically and logically, and find the offending code!
The best way to detect logic errors is to come up with a set of tests
and check the output from the program. A test consists of a set of inputs,
the expected set of outputs and the actual outputs from the program.
If the actual output matches the expected output, then the test passes,
otherwise the test fails and you must try to find the error in your code.
When developing your tests, not only must you test "normal" or "usual" cases,
you must also check "marginal" or "boundary" cases.
For example, suppose you have a program that asks for a user's age and
determines if the user is a senior citizen. Suppose that senior citizens
are classified as people aged 65 or more. When you test the program,
you want to test a value less than 65 and a value more than 65 and make
sure that the program prints "Not senior citizen" and "Is
senior citizen", respectively. These are "regular" cases.
It is also critical to test the value 65. When the input is 65, the output
should indicate that the user is a senior citizen. If the programmer
uses "> 65" instead of ">= 65" in their code,
the output will not be correct. It is a common error to write ">" when ">=" should
be used — this error will be detected only if we test the value
65. We refer to 65 as a "boundary" value.
Suppose you run a test that fails. How do you find the error?
There are various techniques for doing this — you can
simply "walk" through the code checking that each
line is logically correct. With a small piece of code, this
will often work quite well. However, for larger pieces of code
you will want to try to narrow your search down to a smaller
chunk of code. You can do this by inserting
System.out.println(...); statements in your
code. Specifically, you can print out the value of key
variables and ensure that they are correct, rather than only printing out the final, reported values. It might
be helpful to print out some of the intermediate values
computed along the way and check that each of them is
correct. Try using this technique — you will find that
you detect logic errors much more quickly than staring at the
code! (Be sure that you remove your extra println statements before submitting your code, however!)
Specification:
In this part of the assignment, you will write your own Java program, AmateurMagician.java,
impersonating a tongue-tied magician who doesn't have a very impressive
act. The user picks a card, and the magician says what that card is.
Eventually.
Here's an example session with the game:
Welcome to the Amateur Magician Card Tricks Night!
--------------------------------
Pick a card, any card, any card will do
No strings up my sleeve - well, maybe a few
Glease pive... no!
Leasepay ivegay... that's not it!
Pl33s3 g1ve... ok, deep breath...
Pwease give... uh...
Please give
a card name (ace, one-nine, jack, queen, or king): three
a card suit (clubs, diamonds, hearts, spades): diamonds
You picked:
dhree of tiamonds... no!
hreetay of iamondsday... that's not it!
thr33 0f di@m0ndz... ok, deep breath...
dwee of diamonds... uh...
three of diamonds!
Whew. Play again?
(Note that the user's input is in green for clarity. You do not have to make the user's input green in your program!)
Here's a detailed list of tasks for your program:
- Print a banner identifying the game
- Print a brief introduction from the magician
- Print a prompt for the card name
- Read the card name
- Print a prompt for the card suit
- Read the card suit
- Print a message saying "You picked:"
- Print the Spoonerism version of the card designation
- Print the Pig Latin version of the card designation
- Print the LeetSpeak version of the card designation
- Print the Elmer Fudd version of the card designation
- Print the card designation correctly
- Sign off with a final message to the user
For the sanity of the markers, please make all your prompts and output
look as similar as possible to the example above.
You must create the card designation by combining the name and suit,
with the word "of" surrounded by spaces in between them. The rules for
the four ways that the magician gets it wrong are:
Reflection Questions:
Be sure to answer these reflection questions about Part
2 after you finish the part. There are no right answers to these
questions, and we don't expect more than a couple of sentences for each
question. However, we will grade on your effort to answer
throughtfully.
- Did you end up implementing and testing the
assignment piece-by-piece or all-at-once? (You won't be penalized for
either answer!) In either case, what were the biggest frustrations you
encountered with your approach? Which approach (or combination) do you
think you'll use next time?
- Which confused string rules were the
hardest to implement? Why? Propose something (a method, library, or
statement) that Java could provide you that would make that rule easier
to implement.
- OPTIONAL: What was especially
interesting or frustrating about this part of the assignment? What
could we have done to make it better for you?
Hints:
Do not try to write the whole program in one go.
Instead, try writing and testing it bit by bit. For example, you
might follow these steps, ensuring at each step that you have a
working program:
- Create a nearly-empty program shell for
AmateurMagician.java, make sure you can compile and run it.
- You will probably find the Java object String
to be very useful, make sure you are familiar with its methods
replace and substring.
- You will probably find the Java object Scanner
very useful, make sure you are familiar with its method next.
- Make your program just print the game's banner message, and then test your code!
- Print out the signoff message to the user and end the program, and then test your code!
- After the banner message section, ask the user for the card name, and then test your code!
- Read the card name, and then test your code! (You may want to print out the card name you read, just to verify that your code is working correctly.)
- Ask the user for the card suit, and then test your code!
- Read the card suit, and then test your code!
- You may want to break your name and suit strings up into new
smaller strings. For instance, consider splitting a string into two
new strings: one with just the first character and one with all the
rest. Or splitting a string into two different new strings: one with
everything up until the last character, and one with just the last
character.
- You may want to combine your name and suit strings into a longer
strong, with spaces and " of " in between them.
- Create and print out the correct version string. It's the last
thing that should print out, but it's the easiest one to do, so add
that code first, and then test your code!
- Create and print out a Spoonerism version string, and then test your code!
- Create and print out a Pig Latin version string, and then test your code!
- Create and print out an Elmer Fudd version string, and then test your code!
- Create and print out a LeetSpeak version string, and then test your code! This may be
the hardest one, because one of the substitutions should only happen
at the end of a word.
- Test the full program to make sure that you've implemented the complete specification.
- Make sure you're not redoing work unnecessarily. If you find
yourself doing the same operation more than once consider creating
temporary variables.
- Make sure your code is fully commented, and then test your code!
We're not kidding. It's easy to accidentally mess something up when you
think all you're doing is adding comments. (True, comments have no
effect on the function of your code, but what about that accidental
extra character you typed into an identifier name without noticing it?)
- Declare victory and hand in!
When you have difficulty making a piece work, write as small a program
as possible that uses just that piece. That way, you can isolate the
problems you're having in a small, simple piece of code.
Challenges:
Do you feel the need to do more? Here are a few (completely optional!) suggestions:
- Implement another text filter for your poor magician to
stumble over. For example, the Swedish chef
(bork bork bork!).
- Allow the user to enter a number in digits instead of spelled-out English word.
- Implement true pig latin, where you break up the word before the
first vowel instead of just using the second letter. We're doing a
shortcut here.
- Source Header: Please be sure to include the following comment statement at the
top of each source file that you write in this assignment, plus your README.txt file:
/*
Author: <insert your name here>
Ugrad lab login ID: <insert your ugrad lab login ID here>
Date: <insert date here>
By submitting this file, I acknowledge that the person whose name appears
above is the sole author of this file except as acknowledged in the file below.
*/
- What To Submit: In this assignment you must submit exactly three files, it is not
necessary to submit any other work.
- the source file for the program that you corrected in Part 1,
LotteryWinnings.java
- the source file for the program that you wrote in Part 2,
AmateurMagician.java
- the answers to the reflection questions for Parts 1 and 2, in the text file README.txt
You must submit both a hard and soft copy of your assignment and you
must be sure that these two copies are identical. A penalty will be
applied if there is any difference between the hard and soft copy.
- Hardcopy Submission: Print out the
cover page provided in the WebCT Assignments section and fill it out.
Print the three files listed above. Staple the cover
page and your three files together with the cover page on top and hand
it in to the drop-box labelled "CPSC 111 2005W2" in the basement of
the ICICS building.
- Electronic Submission:
- Zip up the three files listed above
into a single file, assign1.zip.
- If you are in the lab, you can do this by storing all the
files in a single directory, select all the files and then
right-click one of the selected files. Choose FilZip from the
pop-up menu and then select Add to "xxx.zip" where xxx
is the name of the file that you right-clicked. You will see that a
new file named xxx.zip has been created in which all the
files that you selected have been zipped up.
- Note: FilZip is available on the HSH CD so you
can install it on your machine at home if you wish to submit your
files from your home machine.
- Submit the zip file to our electronic handin box.
- Open up a web browser and point it to:
http://www.cs.ubc.ca/ugrad/facilities/windows/handin.shtml
- The first time you use handin, you
will have to install a security certificate on your machine
(instructions will be provided on-screen) and then log in using your
computer science undergraduate account ID and password.
- You will then see a page that looks like:
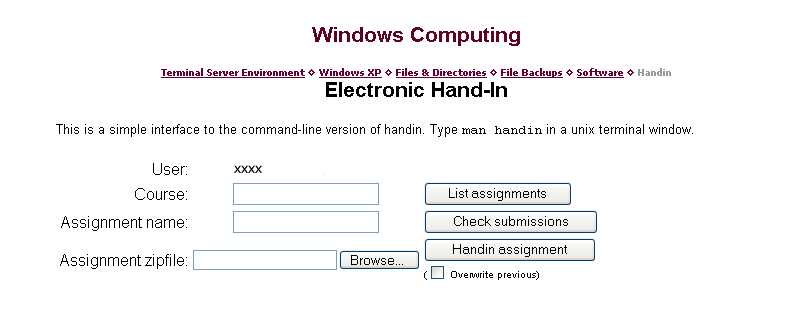
- Enter cs111 for the Course
- Enter assign1 for the Assignment name
- Click the Browse button and select the zip file assign1.zip that contains your assignment files.
- Click the Handin assignment button
- Use 'Save As' in the browser to save a copy of your submission page, just in case there are any problems.
- Important: now check that your work has been submitted correctly by choosing the Check submissions button.
Note that you have to specify the course and assignment name as you did
when you first handed in your assignment. You will see a list of the
files that were contained in your zip file. If any files are missing,
repeat the process.
- You can overwrite your submission any time before the due date by clicking the Overwrite previous box before you click the Handin assignment button.
- If you want to check an assignment due date, select the
List assignments button, and specify the course (cs111).
- Late Penalty:
The following late penalties will be applied if either your hard or soft
copy is not submitted on time. Please note that weekends and holidays are counted!
- 10% deduction if your work is up to 24 hours late
- 30% deduction if your work is up to 48 hours late
- 100% deduction if your work is more than 48 hours late
- A Word of Advice: The demand for our labs tends
to be extremely high on the due date of an assignment. It is not
unusual for students to have to wait hours for work to be printed
because of the enormous queue of print jobs. To avoid last minute
headaches, start early and plan to submit your assignment at least
one day before it is due!
Last modified: Sun Apr 18 16:26:10 PDT 2010